qml.operation¶
This module contains the abstract base classes for defining PennyLane operations and observables.
Warning
Unless you are a PennyLane or plugin developer, you likely do not need to use these classes directly.
See the main operations page for details on available operations and observables.
Description¶
Qubit Operations¶
The Operator
class serves as a base class for operators,
and is inherited by both the Observable
class and the
Operation
class. These classes are subclassed to implement quantum operations
and measure observables in PennyLane.
Each
Operator
subclass represents a general type of map between physical states. Each instance of these subclasses represents eitheran application of the operator or
an instruction to measure and return the respective result.
Operators act on a sequence of wires (subsystems) using given parameter values.
Each
Operation
subclass represents a type of quantum operation, for example a unitary quantum gate. Each instance of these subclasses represents an application of the operation with given parameter values to a given sequence of wires (subsystems).Each
Observable
subclass represents a type of physical observable. Each instance of these subclasses represents an instruction to measure and return the respective result for the given parameter values on a sequence of wires (subsystems).
Differentiation¶
In general, an Operation
is differentiable (at least using the finite-difference
method) with respect to a parameter iff
the domain of that parameter is continuous.
For an Operation
to be differentiable with respect to a parameter using the
analytic method of differentiation, it must satisfy an additional constraint:
the parameter domain must be real.
Note
These conditions are not sufficient for analytic differentiation. For example, CV gates must also define a matrix representing their Heisenberg linear transformation on the quadrature operators.
CV Operation base classes¶
Due to additional requirements, continuous-variable (CV) operations must subclass the
CVOperation
or CVObservable
classes instead of Operation
and Observable
.
Differentiation¶
To enable gradient computation using the analytic method for Gaussian CV operations, in addition, you need to
provide the static class method _heisenberg_rep()
that returns the Heisenberg representation of
the operation given its list of parameters, namely:
For Gaussian CV Operations this method should return the matrix of the linear transformation carried out by the operation on the vector of quadrature operators \(\mathbf{r}\) for the given parameter values.
For Gaussian CV Observables this method should return a real vector (first-order observables) or symmetric matrix (second-order observables) of coefficients of the quadrature operators \(\x\) and \(\p\).
PennyLane uses the convention \(\mathbf{r} = (\I, \x, \p)\) for single-mode operations and observables and \(\mathbf{r} = (\I, \x_0, \p_0, \x_1, \p_1, \ldots)\) for multi-mode operations and observables.
Note
Non-Gaussian CV operations and observables are currently only supported via the finite-difference method of gradient computation.
Contents¶
Operator Types¶
|
Base class representing quantum operators. |
|
Base class representing quantum gates or channels applied to quantum states. |
|
Base class representing observables. |
|
A mixin base class denoting a continuous-variable operation. |
|
Base class representing continuous-variable observables. |
|
Base class representing continuous-variable quantum gates. |
|
Base class for quantum channels. |
|
Container class representing tensor products of observables. |
|
An interface for state-prep operations. |
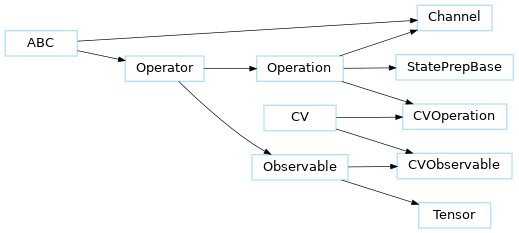
Errors¶
When an Operator
method is undefined, it raises a error type that depends
on the method that is undefined.
Generic exception to be used for undefined Operator properties or methods. |
|
Raised when an Operator’s adjoint version is undefined. |
|
Raised when an Operator’s representation as a decomposition is undefined. |
|
Raised when an Operator’s diagonalizing gates are undefined. |
|
Raised when an Operator’s eigenvalues are undefined. |
|
Exception used to indicate that an operator does not have a generator |
|
Raised when an Operator’s matrix representation is undefined. |
|
Exception used to indicate that an operator does not have parameter_frequencies |
|
Raised when an Operator’s power is undefined. |
|
Raised when an Operator’s sparse matrix representation is undefined. |
|
Raised when an Operator’s representation as a linear combination is undefined. |
Boolean Functions¶
BooleanFn
’s are functions of a single object that return True
or False
.
The operation
module provides the following:
Returns |
|
Returns |
|
Returns |
|
Returns |
|
Returns |
|
Returns |
|
Returns |
|
Returns |
|
Returns |
|
Returns |
Enabling New Arithmetic Operators¶
PennyLane is in the process of replacing Hamiltonian
and Tensor
with newer, more general arithmetic operators. These consist of Prod
,
Sum
and SProd
. By default, using dunder
methods (eg. +
, -
, @
, *
) to combine operators with scalars or other operators will
create the aforementioned newer operators. To toggle the dunders to return the older arithmetic operators,
the operation
module provides the following helper functions:
|
Change dunder methods to return arithmetic operators instead of Hamiltonians and Tensors |
|
Change dunder methods to return Hamiltonians and Tensors instead of arithmetic operators |
Function that checks if the new arithmetic operator dunders are active |
|
Converts |
|
Converts arithmetic operators into a legacy |
Other¶
|
Calculate the derivative of an operation. |
|
Integer enumeration class to represent the number of wires an operation acts on |
An enumeration which represents all wires in the subsystem. |
|
An enumeration which represents any wires in the subsystem. |
PennyLane also provides a function for checking the consistency and correctness of an operator instance.
|
Runs basic validation checks on an |
Operation attributes¶
PennyLane contains a mechanism for storing lists of operations with similar
attributes and behaviour (for example, those that are their own inverses).
The attributes below are already included, and are used primarily for the
purpose of compilation transforms. New attributes can be added by instantiating
new Attribute
objects. Please note that
these objects are located in pennylane.ops.qubit.attributes
, not pennylane.operation
.
Class to represent a set of operators with a certain attribute. |
|
Operations for which composing multiple copies of the operation results in an addition (or alternative accumulation) of parameters. |
|
Operations that are diagonal in the computational basis. |
|
Operations that are generated by a unitary operator. |
|
Operations that are their own inverses. |
|
Operations that support parameter broadcasting. |
|
Operations that are the same if you exchange the order of wires. |
|
Controlled operations that are the same if you exchange the order of all but the last (target) wire. |